How to Build Subscription Management with Supabase
Discover step-by-step guidance on building a subscription management system using Supabase, enhancing your app's functionality with ease and efficiency.
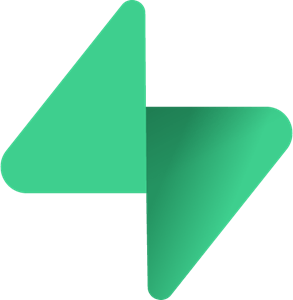
Discover step-by-step guidance on building a subscription management system using Supabase, enhancing your app's functionality with ease and efficiency.
1. Set Up Your Supabase Project
2. Initialize Your Database
3. Install Supabase Client Library
npm install @supabase/supabase-js
4. Initialize Supabase Client
import { createClient } from '@supabase/supabase-js';
const supabaseUrl = 'YOUR_SUPABASE_URL';
const supabaseKey = 'YOUR_SUPABASE_KEY';
const supabase = createClient(supabaseUrl, supabaseKey);
5. Implement Signup and Login Functionality
// Sign up
const { user, session, error } = await supabase.auth.signUp({
email: '[email protected]',
password: 'your-password'
});
// Log in
const { user, session, error } = await supabase.auth.signIn({
email: 'user@example.com',
password: 'your-password'
});
6. Create Subscription Logic
async function createSubscription(userId, plan) {
const { data, error } = await supabase
.from('subscriptions')
.insert([
{ user_id: userId, plan: plan, status: 'active', valid_until: new Date(new Date().setMonth(new Date().getMonth() + 1)) }
]);
if (error) throw error;
return data;
}
7. Manage Subscription Status
async function updateSubscriptionStatus(userId, status) {
const { data, error } = await supabase
.from('subscriptions')
.update({ status: status })
.eq('user\_id', userId);
if (error) throw error;
return data;
}
8. Implement Middleware for Protected Routes
function requireAuth(req, res, next) {
const session = supabase.auth.session();
if (!session) {
return res.status(401).send('Unauthorized');
}
req.user = session.user;
next();
}
9. Frontend (Optional)
// Example code to display subscription plans
const plans = ['Basic', 'Premium', 'Pro'];
plans.forEach(plan => {
const button = document.createElement('button');
button.innerText = plan;
button.onclick = async () => {
const user = supabase.auth.user();
if (user) {
await createSubscription(user.id, plan);
alert('Subscription created successfully!');
} else {
alert('You need to be logged in to subscribe.');
}
};
document.body.appendChild(button);
});
10. Maintain Subscription
const cron = require('node-cron');
cron.schedule('_ _ _ _ *', async () => {
const { data: subscriptions } = await supabase
.from('subscriptions')
.select('*')
.eq('status', 'active');
subscriptions.forEach(async subscription => {
if (new Date(subscription.valid_until) < new Date()) {
await updateSubscriptionStatus(subscription.user_id, 'expired');
}
});
});
Nocode tools allow us to develop and deploy your new application 40-60% faster than regular app development methods.
Save time, money, and energy with an optimized hiring process. Access a pool of experts who are sourced, vetted, and matched to meet your precise requirements.
With the Bootstrapped platform, managing projects and developers has never been easier.
Bootstrapped offers a comprehensive suite of capabilities tailored for startups. Our expertise spans web and mobile app development, utilizing the latest technologies to ensure high performance and scalability. The team excels in creating intuitive user interfaces and seamless user experiences. We employ agile methodologies for flexible and efficient project management, ensuring timely delivery and adaptability to changing requirements. Additionally, Bootstrapped provides continuous support and maintenance, helping startups grow and evolve their digital products. Our services are designed to be affordable and high-quality, making them an ideal partner for new ventures.
Fast Development: Bootstrapped specializes in helping startup founders build web and mobile apps quickly, ensuring a fast go-to-market strategy.
Tailored Solutions: The company offers customized app development, adapting to specific business needs and goals, which ensures your app stands out in the competitive market.
Expert Team: With a team of experienced developers and designers, Bootstrapped ensures high-quality, reliable, and scalable app solutions.
Affordable Pricing: Ideal for startups, Bootstrapped offers cost-effective development services without compromising on quality.
Supportive Partnership: Beyond development, Bootstrapped provides ongoing support and consultation, fostering long-term success for your startup.
Agile Methodology: Utilizing agile development practices, Bootstrapped ensures flexibility, iterative progress, and swift adaptation to changes, enhancing project success.